Get Started with the .NET/C# Driver
On this page
- Overview
- Download the .NET/C# Driver
- Create a Project Directory
- Install the .NET/C# Driver
- Deploy a MongoDB Atlas Cluster
- Create a Free MongoDB Deployment on Atlas
- Save your Credentials
- Create a Connection String
- Find your MongoDB Atlas Connection String
- Copy your Connection String
- Update the Placeholders
- Set an Environment Variable
- Run a Sample Query
- Create your .NET/C# Application
- Run your Application
- Next Steps
Overview
The .NET/C# Driver is a NuGet package that you can use to connect to and communicate with MongoDB. This guide shows you how to create an application that uses the .NET/C# Driver to connect to a MongoDB cluster hosted on MongoDB Atlas.
Tip
MongoDB Atlas is a fully managed cloud database service that hosts your MongoDB deployments. You can create your own free (no credit card required) MongoDB Atlas deployment by following the steps in this guide.
Follow this guide to connect a sample C# application to a MongoDB Atlas deployment. If you prefer to connect to MongoDB using a different driver or programming language, see our list of official drivers.
Download the .NET/C# Driver
After you complete these steps, you have a new .NET framework project and the .NET/C# Driver installed.
Deploy a MongoDB Atlas Cluster
You can create a free tier MongoDB deployment on MongoDB Atlas to store and manage your data. MongoDB Atlas hosts and manages your MongoDB database in the cloud.
Create a Free MongoDB Deployment on Atlas
Complete the Get Started with Atlas guide to set up a new Atlas account and load sample data into a new free tier MongoDB deployment.
After you complete these steps, you have a new free tier MongoDB deployment on Atlas, database user credentials, and sample data loaded in your database.
Create a Connection String
You can connect to your MongoDB deployment by providing a connection URI, also called a connection string, which instructs the driver on how to connect to a MongoDB deployment and how to behave while connected.
The connection string includes the hostname or IP address and port of your deployment, the authentication mechanism, user credentials when applicable, and connection options.
Tip
To connect to a self-managed (non-Atlas) deployment, see Create a MongoClient.
Find your MongoDB Atlas Connection String
To retrieve your connection string for the deployment that you created in the previous step, log into your Atlas account and navigate to the Database section and click the Connect button for your new deployment.
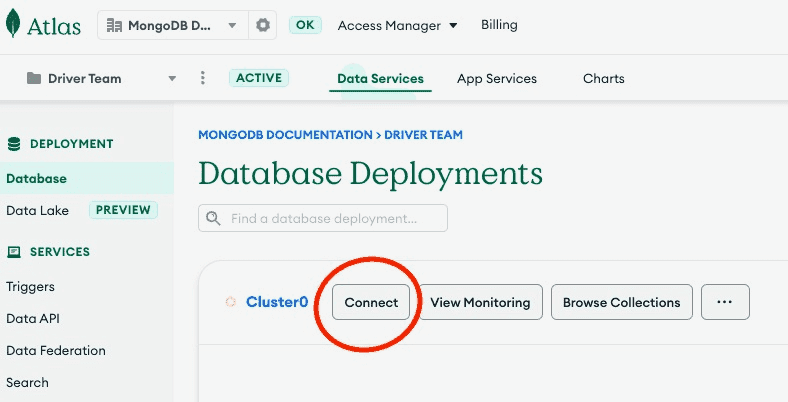
Proceed to the Connect your application section and select "C# / .NET" from the Driver selection menu and the version that best matches the version you installed from the Version selection menu.
Select the Password (SCRAM) authentication mechanism.
Deselect the Include full driver code example option to view the connection string.
Set an Environment Variable
In your shell, run the following code to save your MongoDB
connection string to an
environment variable. Replace <your MongoDB URI>
with the connection
string that you saved to a file in the previous step.
export MONGODB_URI="<your MongoDB URI>"
Note
PowerShell
If you're using Microsoft PowerShell, run the following command instead:
set MONGODB_URI="<your MongoDB URI>"
Storing your credentials in an environment variable is safer than hardcoding them in your source code.
After completing these steps, you have a connection string that contains your database username and password.
Run a Sample Query
Create your .NET/C# Application
Copy and paste the following code into the Program.cs
file in your application:
using MongoDB.Driver; using MongoDB.Bson; var connectionString = Environment.GetEnvironmentVariable("MONGODB_URI"); if (connectionString == null) { Console.WriteLine("You must set your 'MONGODB_URI' environment variable. To learn how to set it, see https://www.mongodb.com/docs/drivers/csharp/current/get-started/create-connection-string"); Environment.Exit(0); } var client = new MongoClient(connectionString); var collection = client.GetDatabase("sample_mflix").GetCollection<BsonDocument>("movies"); var filter = Builders<BsonDocument>.Filter.Eq("title", "Back to the Future"); var document = collection.Find(filter).First(); Console.WriteLine(document);
Run your Application
In your shell, run the following command to start this application:
dotnet run csharp-quickstart.csproj
The output includes details of the retrieved movie document:
{ _id: ..., plot: 'A young man is accidentally sent 30 years into the past...', genres: [ 'Adventure', 'Comedy', 'Sci-Fi' ], ... title: 'Back to the Future', ... }
Tip
If you encounter an error or see no output, ensure that you specified the proper connection string, and that you loaded the sample data.
After you complete these steps, you have a working application that uses the driver to connect to your MongoDB deployment, runs a query on the sample data, and prints out the result.
Next Steps
Congratulations on completing the tutorial!
In this tutorial, you created a C# application that connects to a MongoDB deployment hosted on MongoDB Atlas and retrieves a document that matches a query.
Learn more about the .NET/C# Driver from the following resources:
Learn how to insert documents in the Insert Documents section.
Learn how to find documents in the Read Operations section.
Learn how to update documents in the Update One and Update Many sections.
Learn how to delete documents in the Delete Documents section.
Note
If you run into issues on this step, ask for help in the MongoDB Community Forums or submit feedback by using the Rate this page tab on the right or bottom right side of this page.